Overview
OAuth2 is an authorization protocol that allows users to exchange data with third-party applications, without sharing their password and by limiting access.
The following instructions provide detailed steps to help you use Keyyo APIs.
For more details about OAuth2, refer to the OAuth 2.0 framework RFC 6750.
Server Side
The following workflow shows the process to get an access token the first time (you'll also get a refresh token):
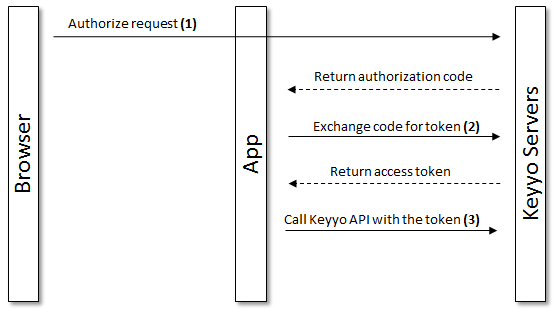
Because an access token expires, when it happens, you need to ask for a new one with the refresh token you got in step 2.
The following workflow shows the process to get a new access token by exchanging your refresh token:
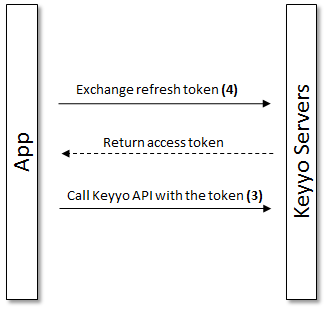
First, you need to register your app before starting.
Get the authorization code (1)
Method | Endpoint |
---|---|
GET |
https://ssl.keyyo.com/oauth2/authorize.php |
Parameters
Name | Required | Description |
---|---|---|
client_id | YES | The client app identifier |
response_type | YES | The value must be set to code |
state | YES | This value contains a unique random string used against CSRF (Cross-Site Request Forgery) attacks |
scopes | NO | The list of permissions separated by commas |
redirect_uri | YES | The callback URL defined in your application settings |
Example
- PHP
$client_id = "Your client identifier";
$keyyo_authorize_endpoint = "https://ssl.keyyo.com/oauth2/authorize.php";
// Redirect the browser to Keyyo's login/authorization form
$_SESSION["auth_state"] = uniqid();
$authorize_url = sprintf("%s?client_id=%s&response_type=code&state=%s&redirect_uri=%s",
$keyyo_authorize_endpoint, $client_id, $_SESSION["auth_state"]),
"https://domain.com/callback");
header("Location: " . $authorize_url);
Response
You will be redirected to your callback URL defined in your application settings.
Exemple: http://www.domain.com/callback.php?code=<Your code>&state=<Your state>
Name | Description |
---|---|
code | The authorization code returned |
state | Your state value |
At this time, you will receive an authorization code and the previous state passed as a parameter in the URL.
Exchange these values to get the refresh token and your first access token.
Warning : If states don't match, it's probably a CSRF attack and the process must be aborted.
Get the access token (2)
Method | Endpoint |
---|---|
POST |
https://api.keyyo.com/oauth2/token.php |
Parameters
Name | Required | Description |
---|---|---|
client_id | YES | The client app identifier |
client_secret | YES | The client app secret |
code | YES | The previous code received |
grant_type | YES | The value must be set to authorization_code |
state | YES | The value must match with the state value of your previous request |
Example
- PHP
$client_id = "<Your client identifier>"
$client_secret = "<Your client secret>"
$keyyo_token_endpoint = "https://api.keyyo.com/oauth2/token.php";
if (!isset($_GET["state"]) || (isset($_GET["state"]) && $_GET["state"] != $_SESSION["auth_state"])) {
header("Content-Type: application/json");
die(json_encode(["error" => "invalid_state", "error_description" => "Invalid state"]));
}
// Send a cURL request using request"s authorization code
$curl = curl_init($keyyo_token_endpoint);
curl_setopt($curl, CURLOPT_POST, true);
curl_setopt($curl, CURLOPT_POSTFIELDS, array(
"client_id" => $client_id,
"client_secret" => $client_secret,
"grant_type" => "authorization_code",
"redirect_uri" => $redirect_uri,
"code" => $_GET["code"]
));
curl_setopt($curl, CURLOPT_RETURNTRANSFER, 1);
$auth_data = curl_exec($curl);
// Retrieve the access token
if ($auth_data === false)
die("cURL request failed");
$response = json_decode($auth_data);
if (is_null($response))
die("Could not parse cURL response body.");
if (isset($response->error))
die(isset($response->error_description) ? $response->error_description : $response->error);
// Output the access token and its lifetime
echo "Your access token is: ", $response->access_token, "<br />";
echo "It expires in: ", $response->expires_in, " seconds <br />";
Response
- PHP
{ "access_token":"<Your access token>",
"expires_in":3600,"token_type":"bearer","scope":"a.user o.w.voipprofile","refresh_token":"<Your refresh token>" }
Name | Description |
---|---|
access_token | An acces token (IMPORTANT: You can use this access token to start consuming the API in step 3 but it will expires, and when it does, see step 4 on how to use the refresh token to obtain a new one.) |
expires_in | The access token expiration (in seconds) |
token_type | Bearer (meaning access_token is generated and send back you) |
scope | The scope list that you have defined in the OAuth authorization page |
refresh_token | The refresh token (IMPORTANT: When your access token expires, see step 4 on how to use the refresh token to obtain a new one.) |
Consume the API with an access token (3)
To use REST Keyyo APIs, we advise you to use Keyyo Manager library available on Github.
For more information, you can refer to its documentation.
Example
- PHP
$access_token = "<Your access token>";
$keyyo_manager = new \Keyyo\Manager\Client("1.0", $access_token);
// Retrieve all services from the authenticated customer
$services = $keyyo_manager->services();
// Loop over their services
foreach ($services as $service) {
// ...
}
Get a new access token with the refresh token (4)
When your access token has expired, you should use your refresh token to get a new one.
Method | Endpoint |
---|---|
POST |
https://api.keyyo.com/oauth2/token.php |
Parameters
Name | Required | Description |
---|---|---|
client_id | YES | The client app identifier |
client_secret | YES | The client app secret |
grant_type | YES | The value must be set to refresh_token |
refresh_token | YES | The refresh token obtained during step 2 |
redirect_uri | YES | The callback URL defined in your application settings |
Example
- PHP
$client_id = "<Your client identifier>";
$client_secret = "<Your client secret>";
$redirect_uri = "https://www.domain.com/callback";
$refresh_token = "<Your refresh token>";
$keyyo_token_endpoint = "https://api.keyyo.com/oauth2/token.php";
// Send a cURL request using request"s authorization code
$curl = curl_init($keyyo_token_endpoint);
curl_setopt($curl, CURLOPT_POST, true);
curl_setopt($curl, CURLOPT_POSTFIELDS, array(
"client_id" => $client_id,
"client_secret" => $client_secret,
"grant_type" => "refresh_token",
"refresh_token" => $refresh_token,
"redirect_uri" => $redirect_uri
));
curl_setopt($curl, CURLOPT_RETURNTRANSFER, 1);
$auth_data = curl_exec($curl);
// Retrieve the access token
if ($auth_data === false)
die("cURL request failed");
$response = json_decode($auth_data);
if (is_null($response))
die("Could not parse cURL response body.");
if (isset($response->error))
die(isset($response->error_description) ? $response->error_description : $response->error);
// Output the access token and its lifetime
echo "Your access token is: ", $response->access_token, "<br />";
echo "It expires in: ", $response->expires_in, " seconds <br />";
Response
- PHP
{ "access_token":"<Your access token>","expires_in":3600,"token_type":"bearer","scope":"a.user o.w.voipprofile" }
Scopes
Scopes limit the access to some parts of the APIs. If you try to access a specific resource without defining the scope in step 1, you will get a "403 Forbidden" error.
You can define one scope or several scopes (separated by commas).
Please refer to the Manager API Documentation for the list of scopes defined for its webservices.
Common errors
Parameters are missing
To get an authorization code you MUST provide 4 parameters as explained in step 1.
- PHP
{
"error": "invalid_request",
"error_description": "Invalid request, parameters are missing"
}
Response type incorrect or missing
To get an authorization code, the response type parameter is required and MUST be set to "code".
- PHP
{
"error": "invalid_request",
"error_description": "The response_type parameter value is missing or incorrect, the value MUST be set to "code""
}
State parameter is missing
If state parameter is not supplied, the request cannot be processed. This parameter is used against CSRF attacks.
- PHP
{
"error": "invalid_request",
"error_description": "The state parameter is required"
}
Invalid client identifier
If your client identifier is incorrect, refer to your application settings to obtain the correct one.
- PHP
{
"error": "invalid_client",
"error_description": "The client id supplied is invalid"
}